Getting the fingerprint of a certificate in Go
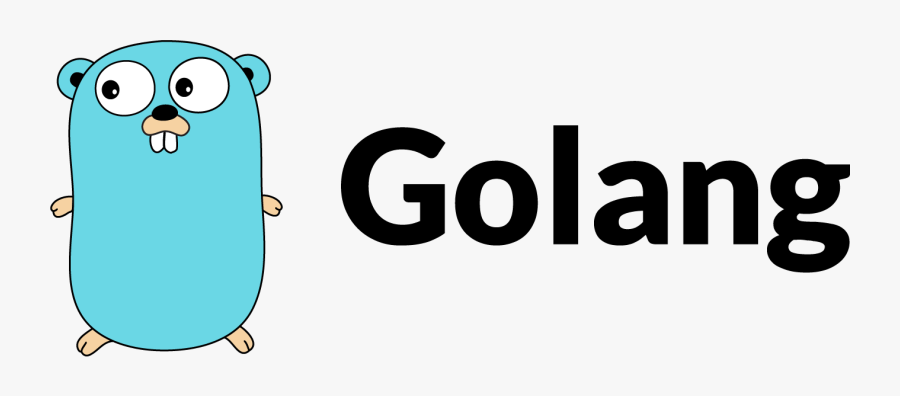
If you need to get the fingerprint for a given certificate, we can use OpenSSL to do it, but we may also want to do the same in Go.
We can adapt this StackOverflow answer and this blog post to produce the following:
package main
import (
"crypto/sha1"
"crypto/tls"
"fmt"
"log"
)
func fingerprint(address string) string {
conf := &tls.Config{
InsecureSkipVerify: true, // as it may be self-signed
}
conn, err := tls.Dial("tcp", address, conf)
if err != nil {
log.Println("Error in Dial", err)
return ""
}
defer conn.Close()
cert := conn.ConnectionState().PeerCertificates[0]
fingerprint := sha1.Sum(cert.Raw)
return fmt.Sprintf("%x", fingerprint) // to make sure it's a hex string
}
func main() {
fmt.Println(fingerprint("google.com:443"))
}