Pretty Printing JSON on the Command Line with Go
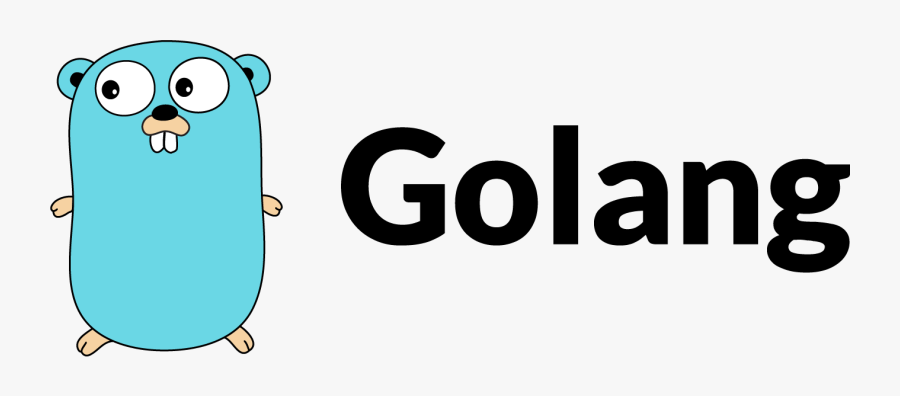
As with other posts in this series, it's useful to be able to quickly convert a JSON string to a pretty-printed version.
In today's installment, we'll do it using Go.
Adapting the example from gosamples.dev, we can use the json.Indent
call to perform the coercion, reading stdin
using io.ReadAll
, resulting in the following:
package main
import (
"bytes"
"encoding/json"
"fmt"
"io"
"os"
"strings"
)
func PrettyString(str []byte) (string, error) {
var prettyJSON bytes.Buffer
if err := json.Indent(&prettyJSON, str, "", " "); err != nil {
return "", err
}
return prettyJSON.String(), nil
}
func main() {
stdin, err := io.ReadAll(os.Stdin)
if err != nil {
panic(err)
}
res, err := PrettyString(stdin)
if err != nil {
panic(err)
}
fmt.Println(strings.TrimSuffix(res, "\n"))
}
This then allows us to convert:
{"key":[123,456],"key2":"value"}
To a pretty output:
go run main.go < file.json