Returning a Value, or a Default, From a Java Optional
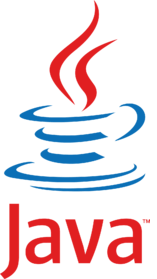
Java Optional
s are great for giving you flexibility over interacting with values that may not be present, and include a means for more safely retrieving values from nullable types.
For instance, you may want to call a method on a maybe-null
object passed into your method, or return a default value:
private static String getName(Principal nullable) {
Optional<Principal> maybePrincipal = Optional.ofNullable(nullable);
if (maybePrincipal.isPresent()) {
return maybePrincipal.get().getName();
} else {
return "<unknown>";
}
}
This is fine, but doesn't follow the functional style that's possible with the Optional
class.
What you may want to do instead is the following (linebreaks added for visibility):
private static String getName(Principal nullable) {
Optional<Principal> maybePrincipal = Optional.ofNullable(nullable);
return maybePrincipal
.map(Principal::getName)
.orElse("<unknown>");
}