Disabling the logging of Spring Security's Default Security Password
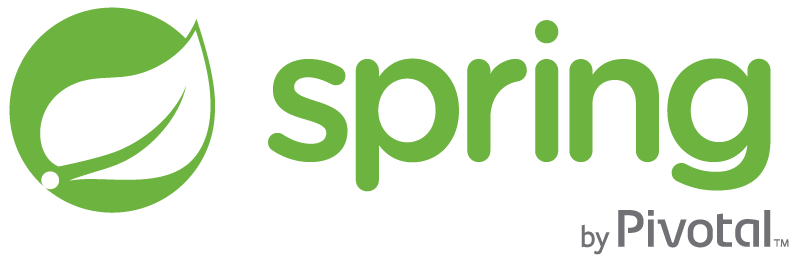
If you use Spring Boot and Spring Security, you may recognise the following from your logs:
2020-06-19 08:54:32.698 INFO 14983 --- [ main] .s.s.UserDetailsServiceAutoConfiguration :
Using generated security password: 2ec7edf2-4fe0-4f25-878e-9f4f50001d06
This security password can be used with the default user, user
, and would allow anyone with access to your logs to be able to authenticate to your service. This is a bad thing!
So how do we stop that? We've got a few options below, in order of my preference. Each of these have been tested with Spring Boot and Spring Security v2.3.1.RELEASE
.
Excluding the autoconfiguration class
To prevent this user from being auto-configured, we can exclude the autoconfiguration class, which means Spring Security won't set up, and log, the default user.
We can do this on our main application class:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration;
@SpringBootApplication(exclude = {UserDetailsServiceAutoConfiguration.class})
@Configuration
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Or via a properties file:
spring.autoconfigure.exclude=org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration
Overriding the AuthenticationManager
Alternatively, we can follow Stefan's comment on Remove “Using default security password” on Spring Boot:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class MyCustomSecurityConfig extends WebSecurityConfigurerAdapter { // via https://stackoverflow.com/a/41856630/2257038
@Override
protected void configure(AuthenticationManagerBuilder authManager) throws Exception {
// This is the code you usually have to configure your authentication manager.
// This configuration will be used by authenticationManagerBean() below.
}
@Bean
public AuthenticationManager authenticationManagerBean() throws Exception {
// ALTHOUGH THIS SEEMS LIKE USELESS CODE,
// IT'S REQUIRED TO PREVENT SPRING BOOT AUTO-CONFIGURATION
return super.authenticationManagerBean();
}
}
By providing a custom bean that overrides the AuthenticationManagerBuilder
, we can replace the autogenerated beans with our own, which would mean that UserDetailsServiceAutoConfiguration
doesn't get triggered.
Disabling logging for that class
Alternatively, if we want it to be autoconfigured, but not logged, we can change the logging configuration for that class:
# completely remove it
logging.level.org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration=OFF
# or only log WARN and above
logging.level.org.springframework.boot.autoconfigure.security.servlet.UserDetailsServiceAutoConfiguration=WARN