Generate Plain Old Java Objects (POJOs) from XML Schema Definitions with xjc
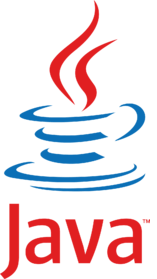
Yesterday I was fighting with an incredibly annoying issue with Jackson's XML extension suddenly deciding to stop parsing my site's sitemap.xml.
While endlessly trawling through Stack Overflow posts, I found this Stack Overflow answer which mentioned the use of the command xjc
, which is bundled in the JDK!
This command takes a given XSD file and generates the given Java classes from that schema, even from a URL:
$ xjc https://www.sitemaps.org/schemas/sitemap/0.9/sitemap.xsd
parsing a schema...
compiling a schema...
org/sitemaps/schemas/sitemap/_0/ObjectFactory.java
org/sitemaps/schemas/sitemap/_0/TChangeFreq.java
org/sitemaps/schemas/sitemap/_0/TUrl.java
org/sitemaps/schemas/sitemap/_0/Urlset.java
org/sitemaps/schemas/sitemap/_0/package-info.java
I thought this was pretty great for folks who work with XSD-defined content types, not least because the generated class is pretty nice, with a fair few comments and a nice interface to the POJO:
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.8-b130911.1802
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2020.02.03 at 08:40:05 PM GMT
//
package org.sitemaps.schemas.sitemap._0;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAnyElement;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
*
* Container for the data needed to describe a document to crawl.
*
*
* <p>Java class for tUrl complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="tUrl">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="loc" type="{http://www.sitemaps.org/schemas/sitemap/0.9}tLoc"/>
* <element name="lastmod" type="{http://www.sitemaps.org/schemas/sitemap/0.9}tLastmod" minOccurs="0"/>
* <element name="changefreq" type="{http://www.sitemaps.org/schemas/sitemap/0.9}tChangeFreq" minOccurs="0"/>
* <element name="priority" type="{http://www.sitemaps.org/schemas/sitemap/0.9}tPriority" minOccurs="0"/>
* <any namespace='##other' maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "tUrl", propOrder = {
"loc",
"lastmod",
"changefreq",
"priority",
"any"
})
public class TUrl {
@XmlElement(required = true)
@XmlSchemaType(name = "anyURI")
protected String loc;
protected String lastmod;
@XmlSchemaType(name = "string")
protected TChangeFreq changefreq;
protected BigDecimal priority;
@XmlAnyElement(lax = true)
protected List<Object> any;
/**
* Gets the value of the loc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLoc() {
return loc;
}
/**
* Sets the value of the loc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLoc(String value) {
this.loc = value;
}
/**
* Gets the value of the lastmod property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastmod() {
return lastmod;
}
/**
* Sets the value of the lastmod property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastmod(String value) {
this.lastmod = value;
}
/**
* Gets the value of the changefreq property.
*
* @return
* possible object is
* {@link TChangeFreq }
*
*/
public TChangeFreq getChangefreq() {
return changefreq;
}
/**
* Sets the value of the changefreq property.
*
* @param value
* allowed object is
* {@link TChangeFreq }
*
*/
public void setChangefreq(TChangeFreq value) {
this.changefreq = value;
}
/**
* Gets the value of the priority property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPriority() {
return priority;
}
/**
* Sets the value of the priority property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setPriority(BigDecimal value) {
this.priority = value;
}
/**
* Gets the value of the any property.
*
* <p>
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a <CODE>set</CODE> method for the any property.
*
* <p>
* For example, to add a new item, do as follows:
* <pre>
* getAny().add(newItem);
* </pre>
*
*
* <p>
* Objects of the following type(s) are allowed in the list
* {@link Object }
*
*
*/
public List<Object> getAny() {
if (any == null) {
any = new ArrayList<Object>();
}
return this.any;
}
}
You can also handle this with the Mojohaus jaxb2-maven-plugin.