Extracting Request Parameters Dynamically for a multipart/form-data
Request
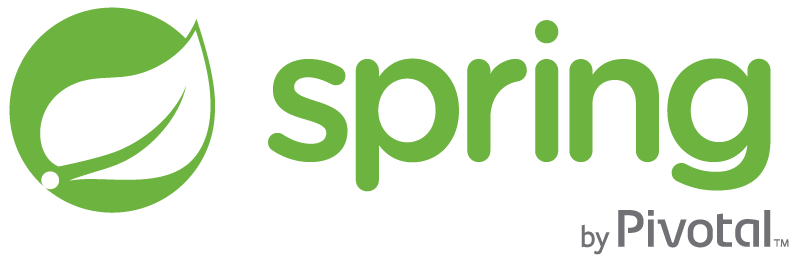
While working on my Micropub endpoint I found that I needed to support multipart/form-data
requests. Having never worked with them, there was a bit of a learning curve, especially around how to get the request parameters out of them (as I was not yet needing to support the media aspect of the request).
I had to dynamically parse these fields out because on any given request there could be anywhere between 4 and 10 parameters in a given request, and I'd not really ever know what that request would look like until receiving it.
Unfortunately most of the articles/answers on Stack Overflow I could find were not very helpful as they were based on the expectation that you had knowledge of which fields you were going to receive.
I did, however, manage to finally track down this StackOverflow answer which mentions you can use the MultipartHttpServletRequest
.
By adding this parameter to your endpoint's method call, you then get access to the full key-value parameters for the request in a Map
which allows you to perform more automated traversal through the request's parameters.
This can be hooked in as such:
import org.springframework.util.MultiValueMap;
@PostMapping(path = "/endpoint", consumes = MediaType.MULTIPART_FORM_DATA_VALUE)
public ResponseEntity<String> multipartForm(MultipartHttpServletRequest multipartRequest) {
Map<String, String[]> multipartRequestParams = multipartRequest.getParameterMap();
// iterate through the Map as you want, or get individual fields out
String[] contentArr = multipartRequestParams.get("content");
// or
String[] contentArr = multiPartRequest.getParameter("content");
}
This has been tested with Spring Boot 2.1.4.RELEASE (which contains Spring 5.1.6.RELEASE).