Parsing Key-Value URL Fragments with Node.JS
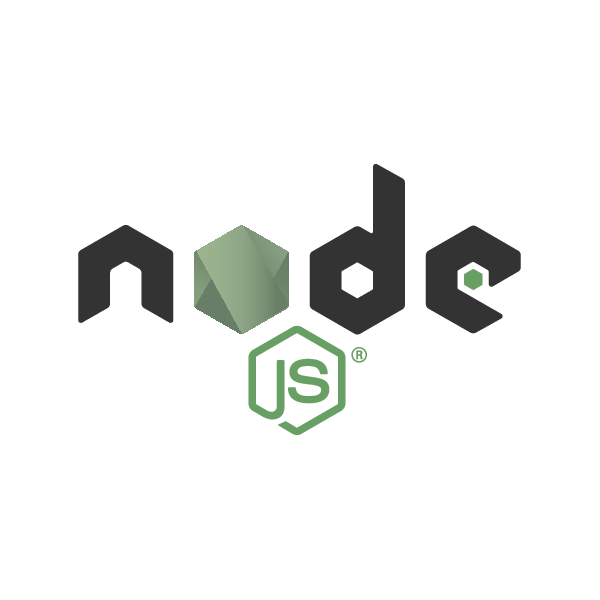
Although the URL fragment isn't defined explicitly as a key-value pair, there are times where it may be, for instance during the OAuth2 Implicit Grant or the OpenID Connect Hybrid Flow.
We can use the url
module to actually parse our URL out, and then the querystring
module to extract the key-value parameters for the fragment, instead of hand-rolling our own:
const url = require('url');
const querystring = require('querystring');
const theUrl = 'https://example.com/auth/callback#code=foo&state=blah';
var fragmentParams = querystring.parse(url.parse(theUrl).hash.replace('#', ''));
console.log(fragmentParams);
// Object: null prototype] { code: 'foo', state: 'blah' }
console.log(JSON.stringify(fragmentParams, null, 4));
/*
{
"code": "foo",
"state": "blah"
}
*/
Also note that yes, this should be received by your browser, not by some Node code which runs on the backend, but for the purpose I've been testing it with, the Node code for it was important.
And for a handy one-liner, which will let you optionally specify the parameter to display:
# note the required use of `--` at the end!
$ echo 'https://example.com/auth/callback#code=foo&state=blah' | node -r querystring -r url -e 'q = querystring.parse(url.parse(fs.readFileSync("/dev/stdin", "utf-8")).hash.replace("#", ""));console.log((process.argv.slice(0)[1]) ? (q[process.argv.slice(0)[1]] || "") : JSON.stringify(q, null, 4));' -- code